Mastering String Handling in JavaScript: Efficient Looping Techniques
Written on
Understanding Strings in JavaScript
Strings are a vital data type in JavaScript, and efficient manipulation of these strings is essential for numerous programming tasks. A frequent operation involves iterating through strings to access and process individual characters. In this guide, we will explore various methods and strategies for mastering string iteration in JavaScript.
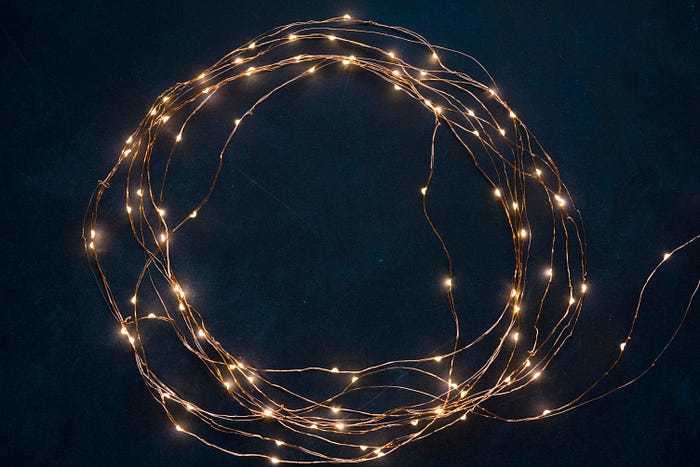
Exploring String Basics in JavaScript
Before we dive into string iteration techniques, it's important to understand the nature of strings in JavaScript. A string is defined as a sequence of characters, which can be enclosed in single quotes (''), double quotes (""), or backticks (``). Notably, strings are immutable, indicating that once they are created, their content cannot be altered.
Looping Through Strings with a Traditional For Loop
A fundamental method to iterate over a string in JavaScript is by utilizing a traditional for loop. This approach allows you to access each character in the string and perform necessary operations on them.
const str = "Hello, World!";
for (let i = 0; i < str.length; i++) {
console.log(str[i]);
}
In this example, we employ a for loop to traverse each character of the string str and print it to the console. By using the index i, we can manipulate or extract details from the string as required.
Looping Through Strings with a For...of Loop
A more contemporary and streamlined method for string iteration is the for...of loop. This loop allows for direct access to the characters in a string without requiring an index.
const str = "Hello, World!";
for (const char of str) {
console.log(char);
}
The for...of loop simplifies the iteration process by enabling direct access to each character without the need for an index variable.
Utilizing Array Methods for String Iteration
Another method to loop through strings involves converting them into arrays and utilizing array methods such as forEach, map, or reduce.
const str = "Hello, World!";
Array.from(str).forEach(char => {
console.log(char);
});
By transforming the string into an array via Array.from(), we can apply array methods to iterate over each character effectively.
The first video, JavaScript for Beginners — String Manipulation, provides an excellent foundation in string handling techniques. It covers various methods to manipulate strings effectively.
The second video, BEGINNER Javascript Practice - Loops & Iteration - Histogram Character Count, offers practical exercises for beginners to practice loops and string manipulation skills.
Conclusion
Mastering the techniques for looping through strings in JavaScript is crucial for any programmer aiming to work proficiently with text data. By understanding the various methods—such as traditional for loops, for...of loops, and array methods—you can select the most appropriate strategy for your specific needs.
Engage with these techniques and experiment across different scenarios to enhance your proficiency in string manipulation.